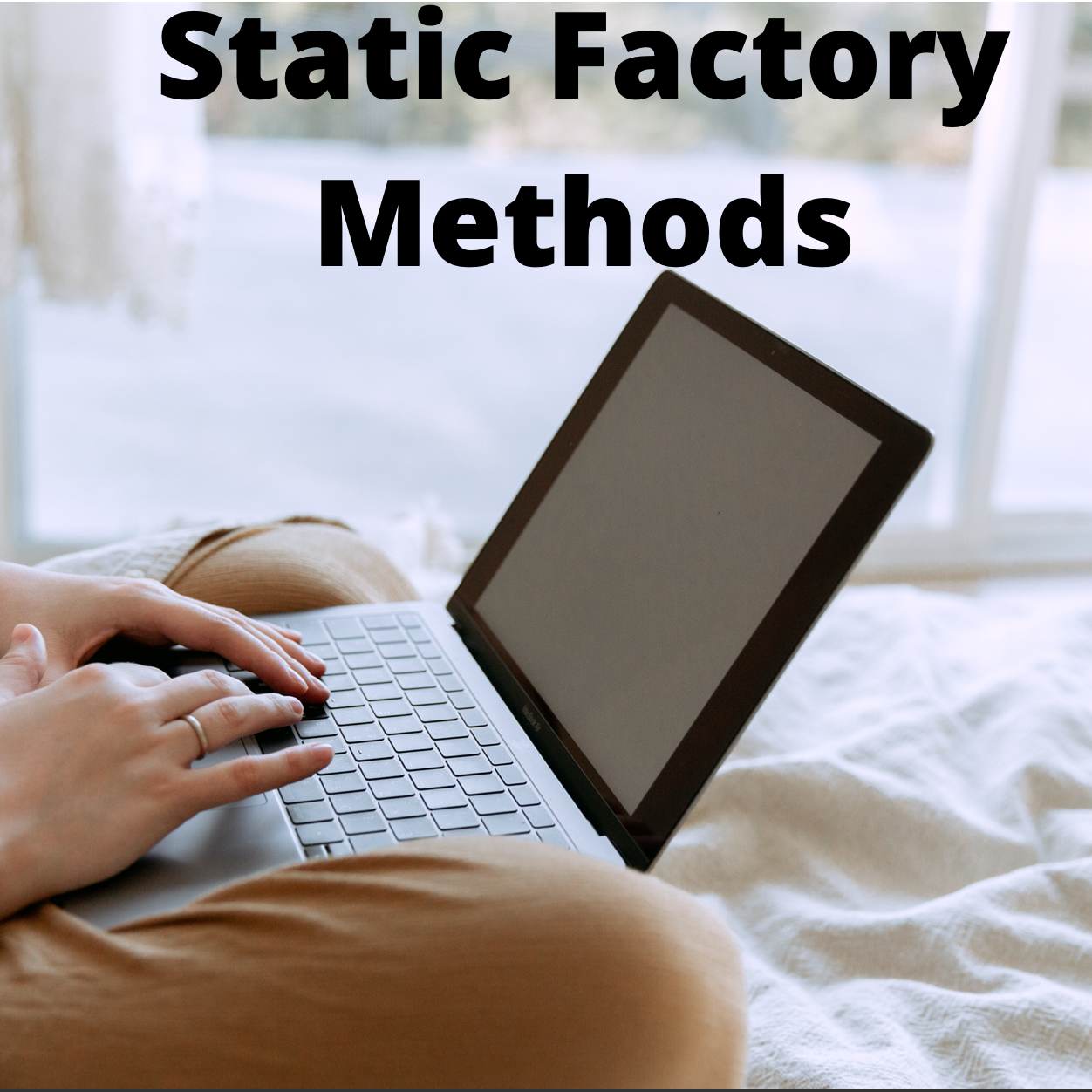
19 Jul Consider static factory methods instead of constructors when creating objects
What is the most common way to create an object of a class? Yeah, you probably guessed it right! By using a constructor. But is it always the best way? We’ll find out here!
Another way to create objects of a class is by using a public static factory method. This method creates an instance of a class and then returns it. Providing a static method has its own set of advantages and disadvantages.
Let us first discuss the advantages:
- The most important advantage of using a static factory method is that it can have a proper name. You don’t have to check the documentation every time to know what is the constructor you should use if you need a particular type of object. Consider the example
BigInteger
constructorBigInteger(int, int, Random)
which is expected to return a prime number. This could be better expressed by using a function namecreateProbablePrime
. A class can have only one constructor with a particular signature. Programmers tend to have a workaround where the order of parameters is different for constructors that behave differently. This is a bad practice since the caller class might call the wrong constructor when there are multiple such permutations of the same set of parameters. - Another advantage of using static factory methods is that they can cache the objects once created and need not create a new object every single time they are invoked like a constructor. This practice often improves the performance and makes the program light weight to run. This is generally used when dealing with classes where the object creation is a costly affair.
- Static factory methods can also return any subtype of their return type. This is something which cannot be achieved by constructors. The return types can also vary as according to the set of parameters provided as input to the factory method which gives great flexibility.
Now let us come to the disadvantages:
- One of the major disadvantages is that the classes that do not have a public or protected constructor cannot be subclassed further. However, this also propagates the idea of preferring composition over inheritance which is considered a good practice.
- Another disadvantage of considering static factory methods is that they are hard to find for the programmer. This can be avoided by using proper naming conventions like
getInstance()
,valueOf()
etc.
Time for some code probably?
Consider the Student class given below:
public class Student {
private final String name;
private final String branch;
private final String yearOfStudy;
public User(String name, String branch, String yearOfStudy) {
this.name = name;
this.branch = branch;
this.yearOfStudy = yearOfStudy;
}
}
When you take a first look there is nothing wrong with the constructor. But when a student takes admission into a university we would want the the yearOfStudy
to be set as First
, wouldn’t we? Now this is where the static factory method comes to your rescue.
public static Student createFreshmanStudent(String name, String branch) {
return new Student(name, branch, "First");
}
And we can create an object of the Student
by using the code below,
Student student = Student.createFreshmanStudent(“John Doe”, “Computer Science”);
That will be all folks! Until the next time 🙂
Sources:
- Effective Java by Joshua Bloch
- And a Google search obviously!
No Comments