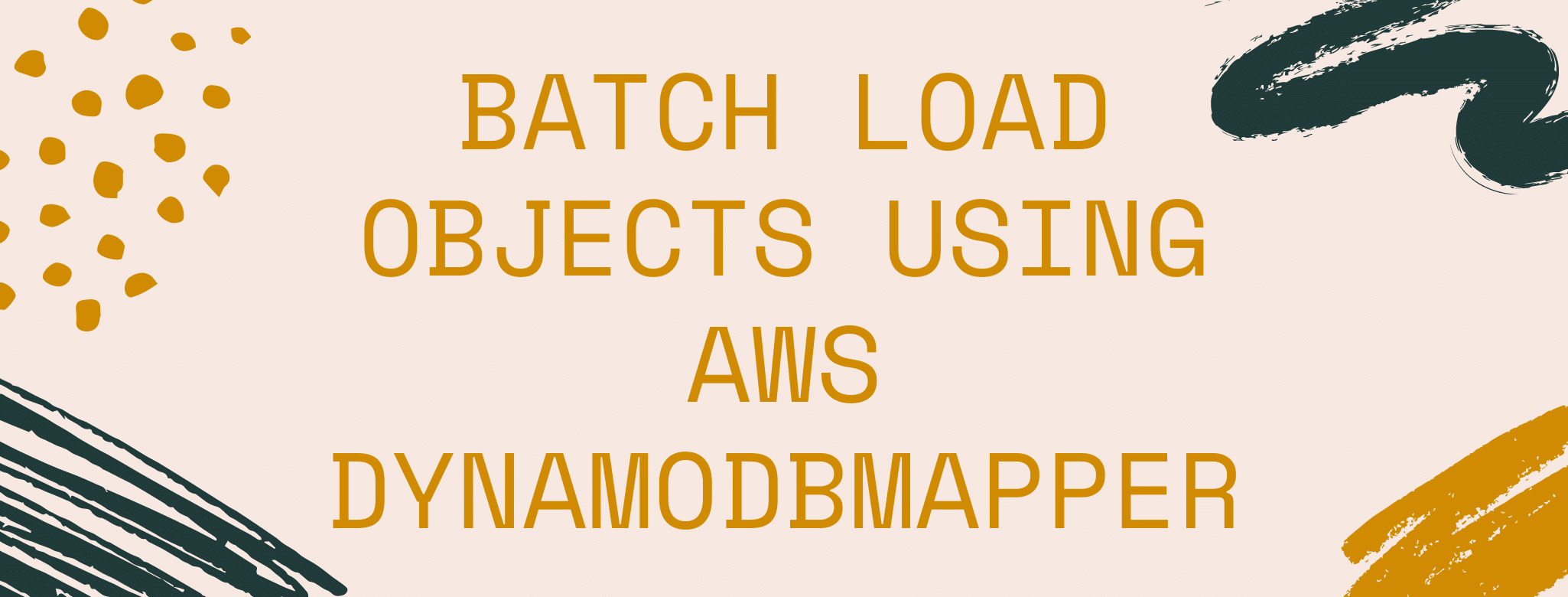
24 Jul Batch load objects using AWS dynamoDBMapper in JAVA
DynamoDbMapper is an amazing class to abstract out a lot of operations for AWS DynamoDb. But this library is not well documented. Here is an example of how to batchLoad objects using dynamoDBMapper in JAVA
List<ArticlesDao> getArticleById(List<LikeDao> likeDaos) { List<ArticlesDao> articlesDaoList = new ArrayList<>();
//The primary key for ArticleDao is "articleId"
for (LikeDao likeDao : likeDaos) {
articlesDaoList.add(ArticlesDao.builder().articleId(likeDao.getArticleId()).build());
}
//Batch Load this list of objects
Map<String, List<Object>> batchResult = dynamoDBMapper.batchLoad(articlesDaoList);
if (batchResult.containsKey(ArticlesDao.TABLE_NAME)
&& batchResult.get(ArticlesDao.TABLE_NAME) != null) {
List<Object> articles = batchResult.get(ArticlesDao.TABLE_NAME);
//Convert the list of Objects into "ArticlesDao
@SuppressWarnings("unchecked")
List<ArticlesDao> articlesDaos = (List<ArticlesDao>) (List<?>) articles;
return articlesDaos;
}
return null;
}
In the above code we are creating a ArrayList, you can also create a HashMap with the object “key” as the hash key for the HashMap.
Map<String, ArticlesDao> getArticlesById(List<LikeDao> likeDaos) {
Map<String, ArticlesDao> articlesDaoMap = new HashMap<>();
List<ArticlesDao> articlesDaoList = new ArrayList<>();
//The primary key for ArticleDao is "articleId"
for (LikeDao likeDao : likeDaos) {
articlesDaoList.add(ArticlesDao.builder().articleId(likeDao.getArticleId()).build());
}
//Batch Load this list of objects
Map<String, List<Object>> batchResult = dynamoDBMapper.batchLoad(articlesDaoList);
if (batchResult.containsKey(ArticlesDao.TABLE_NAME)
&& batchResult.get(ArticlesDao.TABLE_NAME) != null) {
List<Object> articles = batchResult.get(ArticlesDao.TABLE_NAME);
for (Object object : articles) {
ArticlesDao articlesDao = (ArticlesDao) object;
articlesDaoMap.put(articlesDao.getArticleId(), articlesDao);
}
return articlesDaoMap;
}
return null;
}
No Comments